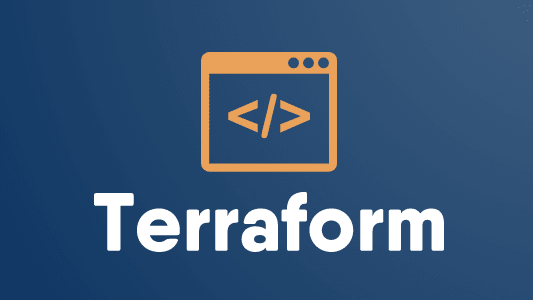
Terraform HCL Intro 3: Conditional Logic
In this post, we’ll cover how to perform conditional logic with Terraform. It’ll be a little weird looking for those who are not used to the Terraform declarative syntax. There are only a few ways to do conditional logic with Terraform:
- Ternary: This is useful for expressions with conditional return values types like String, List, Map, etc. We’ll cover a few examples since the syntax has some caveats.
- Count: This is commonly brought up as a way to perform conditional logic with Terraform. It’s how you conditionally create a resource itself.
Tenary: Simple Starter Examples
We’ll start with simple ternary examples. Note, we’re assigning value here. So the single equal sign (= vs ==) is not a typo.
output "a1" {
value = true ? "is true" : "is false"
}
output "a2" {
value = false ? "is true" : "is false"
}
output "a3" {
value = 1 == 2 ? "is true" : "is false"
}
Running terraform apply
results in:
$ terraform apply -auto-approve
Outputs:
a1 = is true
a2 = is false
a3 = is false
$
Ternary: Example with Functions
Here are some slightly more complicated examples using Terraform functions in the conditional expressions.
output "b1" {
value = contains(["a","b","c"], "d") ? "is true" : "is false"
}
output "b2" {
value = keys({a: 1, b: 2, c: 3}) == ["a","b","c"] ? "is true" : "is false"
}
output "b3" {
value = contains(keys({a: 1, b: 2, c: 3}), "b") ? "is true" : "is false"
}
Running terraform apply
results in:
$ terraform apply -auto-approve
Outputs:
b1 = is false
b2 = is true
b3 = is true
$
Ternary: Multi-line Expression Caveat
One caveat with the ternary operation is single-line vs. multi-line expressions. The ternary operator works well with a single line.
output "c1" {
value = contains(["a","b","c"], "d") ? "is true" : "is false"
}
However, if we try to write the code with multiple lines, Terraform has problems compiling the syntax.
output "c2" {
value = contains(["a","b","c"], "d")
? "is true"
: "is false"
}
The syntax error is something like this:
Error: Argument or block definition required
on outputs.tf line 26, in output "c2":
26: ? "is true"
An argument or block definition is required here.
This is fixed by wrapping the multi-line ternary expressions with (...)
. Example:
output "c3" {
value = (
contains(["a","b","c"], "d")
? "is true"
: "is false"
)
}
I wished someone pointed out to me when first working with HCL, so I’m pointing it out for others to spare the time from figuring out.
Count Technique: Simple Examples
This may be the most common conditional Terraform technique people talk about since it can be used to create a resource conditionally. Count is really a looping construct, but we can abuse it to perform conditional logic.
First, we’ll look an an example that creates a resource:
variable "create1" {
default = true
}
resource "random_pet" "pet1" {
count = var.create1 ? 1 : 0
length = 2
}
output "pet1" {
value = random_pet.pet1
}
This results in:
$ terraform apply -auto-approve
Outputs:
pet1 = [
{
"id" = "superb-tiger"
"length" = 2
"separator" = "-"
},
]
The key here is var.create1
. When it is set to true, then count = 1
, and this results in Terraform creating the random_pet resource. This is how you conditionally create resources with Terraform.
Here’s an example when the ternary condition is false:
variable "create2" {
default = false
}
resource "random_pet" "pet2" {
count = var.create2 ? 1 : 0
length = 2
}
output "pet2" {
value = random_pet.pet2
}
This results in:
$ terraform apply -auto-approve
Outputs:
pet2 = []
In this case, the resource is not created because count = 0
.
An interesting aspect of using the Terraform count attribute is that the result of the resource declaration is no longer a single element. It’s an Array. This can be more clearly seen in the last example output with pet2 = []
. This is useful info to note for the next post in this tutorial series.
Summary
In this post, we covered how to do conditional logic with Terraform. Conditional logic with the ternary operator is pretty straightforward. A ternary caveat is to make sure to wrap multi-line usage of it with parenthesis. The other commonly talked about conditional technique uses the count attribute. It’s how we can create resources conditionally. In the next post, we’ll start to cover loops in Terraform, , we’ll cover conditionals: Terraform HCL Intro 4: Loops with Count and For Each.
The source code for the examples is available for BoltOps Learn Subscribers: terraform-hcl-tutorials/3-conditionals
Want It to be Easier to Work with Terraform?
Check out Terraspace: The Terraform Framework.
The Terraform HCL Language Intro Tutorials
Thanks for reading this far. If you found this article useful, I'd really appreciate it if you share this article so others can find it too! Thanks 😁 Also follow me on Twitter.
Got questions? Check out BoltOps.
You might also like
More tools:
-
Kubes
Kubes: Kubernetes Deployment Tool
Kubes is a Kubernetes Deployment Tool. It builds the docker image, creates the Kubernetes YAML, and runs kubectl apply. It automates the deployment process and saves you precious finger-typing energy.
-
Jets
Jets: The Ruby Serverless Framework
Ruby on Jets allows you to create and deploy serverless services with ease, and to seamlessly glue AWS services together with the most beautiful dynamic language: Ruby. It includes everything you need to build an API and deploy it to AWS Lambda. Jets leverages the power of Ruby to make serverless joyful for everyone.
-
Lono
Lono: The CloudFormation Framework
Building infrastructure-as-code is challenging. Lono makes it much easier and fun. It includes everything you need to manage and deploy infrastructure-as-code.