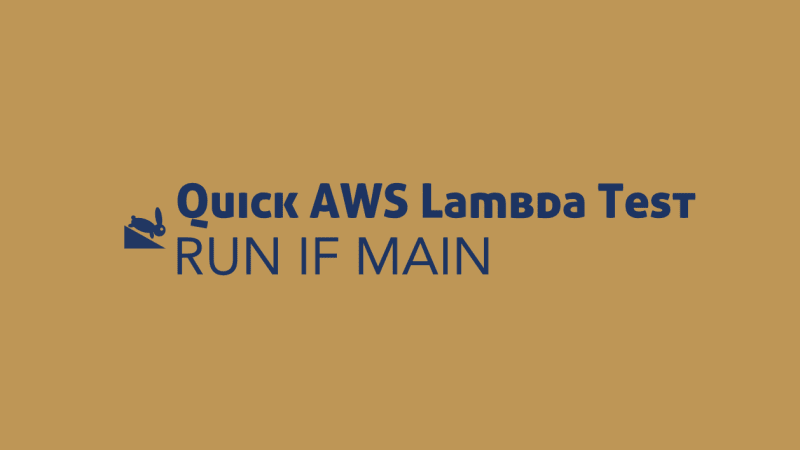
Quick AWS Lambda Testing If Main Script Trick: Ruby, Python, Node, Bash
A quick way to test a Lambda function, is to add a little portion of code at the bottom of the script that tells it to run itself when it is the main script. The if statement means that the script will only run if it is ran directly vs being required as a library.
hi.rb:
if __FILE__ == $0
puts "this script was ran directly"
end
So this will run the if statement:
$ ruby hi.rb # run directly
this script was ran directly
$
And this will not run the if statement:
myfile.rb:
require_relative "hi" # being required, so not ran directly
$ ruby myfile.rb # will not run script since `hi.rb` is being required
$
Always forget the way to do it between languages, so writing it down for posterity.
Here are different ways to check if the script is being ran directly in: Ruby, Python, Node and Bash.
Code Examples
Ruby
def handle(event:, context:)
p event
end
if __FILE__ == $0
event = {hi: "world"}
handle(event: event, context: {})
end
Output:
{:hi=>"world"}
Python
def lambda_handler(event, context):
print(event)
if __name__ == "__main__":
event = {'hi': 'world'}
lambda_handler(event, {})
Output:
$ python hi.py
{'hi': 'world'}
Node
const handler = function(event, context, callback) {
console.log(event);
}
if (require.main === module) {
var event = {'hi': 'world'};
var context = {'fake': 'context'};
exports.handler(event, context);
}
Output:
$ node hi.js
{ hi: 'world' }
Bash
function handler () {
EVENT_DATA=$1
echo "$EVENT_DATA" 1>&2;
RESPONSE="Echoing request: '$EVENT_DATA'"
echo $RESPONSE
}
if [[ "${BASH_SOURCE[0]}" == "${0}" ]]; then
handler '{"test": 1}'
# handler "$@"
fi
Output:
$ node hi.js
{ hi: 'world' }
Thanks for reading this far. If you found this article useful, I'd really appreciate it if you share this article so others can find it too! Thanks 😁 Also follow me on Twitter.
Got questions? Check out BoltOps.
You might also like
More tools:
-
Kubes
Kubes: Kubernetes Deployment Tool
Kubes is a Kubernetes Deployment Tool. It builds the docker image, creates the Kubernetes YAML, and runs kubectl apply. It automates the deployment process and saves you precious finger-typing energy.
-
Jets
Jets: The Ruby Serverless Framework
Ruby on Jets allows you to create and deploy serverless services with ease, and to seamlessly glue AWS services together with the most beautiful dynamic language: Ruby. It includes everything you need to build an API and deploy it to AWS Lambda. Jets leverages the power of Ruby to make serverless joyful for everyone.
-
Lono
Lono: The CloudFormation Framework
Building infrastructure-as-code is challenging. Lono makes it much easier and fun. It includes everything you need to manage and deploy infrastructure-as-code.