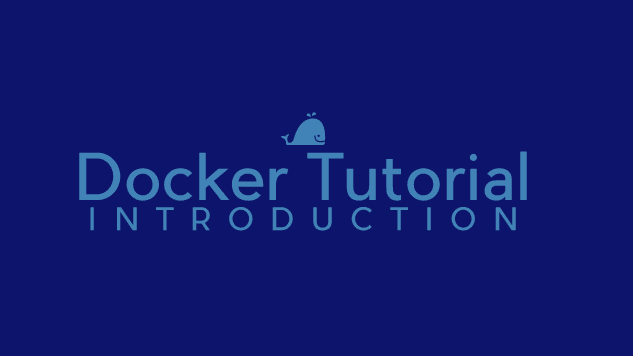
Docker Intro Tutorial: Common Commands Crash Course
Docker is kind of like git
in a sense that there are only a few commands to learn to for it be useful. This tutorial focuses on the docker commands that I find myself most commonly use. This article assumes that you have a general idea of what docker is and have it installed and set up already. Hopefully, it serves as handy reference or cheatsheet for useful common docker commands.
Summary
First, we’ll run through the lifecycle commands:
docker run # starts a new container
docker ps # shows running containers
docker exec # hops or "ssh" into a container
docker stop # stops a container
docker rm # removes a stopped container
docker start # start back up a stopped container
Then we’ll cover the registry commands:
docker build # builds an image from a Dockerfile
docker images # lists the pulled or built images
docker push # pushes the image to a docker registry
Last, we’ll show some combo cleanup commands to help with cleaning containers and images.
For this guide, we’ll use a simple Dockerfile that installs apache and some tools to help with debugging. The project is available at boltops-learn/docker-tutorial. The docker image has also been pushed to Dockerhub at boltops/docker-tutorial
Lifecycle Commands: Docker Run
docker run
docker run
is the main command we can use to start a docker container. Let’s start a container now and poke around:
$ docker run --rm -ti boltops/docker-tutorial bash
bash-4.2# httpd -v
Server version: Apache/2.4.6 (Amazon Linux 2)
Server built: Dec 12 2017 18:43:44
bash-4.2# pwd
/
bash-4.2# ls /etc/httpd/
conf conf.d conf.modules.d logs modules run
bash-4.2#
The command above starts a docker container and puts you into a bash command. I then explored around a bit. When I’m jumping into bash shell to explore, I find myself using these docker run options: --rm -ti
. Let’s cover the options and their purposes:
Option | Purpose |
---|---|
–rm | Once you exit from the container the docker container will automatically be removed. Without this option, what ends up happening after playing with a few containers is that you’ll end up with a bunch of “Exited” containers that show up with docker ps -a . It’s easy to remove the Exited containers but the --rm will automatically clean house for you. |
-ti | This is 2 options combined. -t for tty and -i for interactive. This makes it so the bash shell you’re launching behave more like a normal command shell. |
To exit out of the docker container bash shell. Just run exit
or hit ctrl-D
like you normally would.
Sometimes instead of starting a bash shell, I start the Docker container and let it run its default CMD command. Usually, it starts a webserver and exposes the port to listen on. In these cases, I run this command:
$ docker run --rm -P -d boltops/docker-tutorial
a2b93575b0215405dce221d6004ed34d46530fa6d956600241de0fe38f5f7a55
Let’s cover the new options:
Option | Purpose |
---|---|
-P | -P is short for the --publish-all option. This means docker published any EXPOSE port with the docker image automatically so you can access the port from the host. If apache is listening to port 80, docker will map it to a random port in the ephemeral range. |
-d | -d is short for the --detach option. Docker detaches the container process and run it in the background. |
Notice that the run command with the -d flag returned the docker container id and runs the container in the background. We’ll see the short version of the container in the next command.
docker ps
Leave the docker container running above in the background. You can check for running docker containers with the docker ps
command:
$ docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
a2b93575b021 boltops/docker-tutorial "/usr/sbin/httpd -DF…" 14 seconds ago Up 14 seconds 0.0.0.0:32768->80/tcp mystifying_yonath
Notice, that the first column contains a short version of the container id: a2b93575b021 A user-friendly version of the container is also provided. It’s mystifying_yonath
in this case. The container id or name is used for the next command.
docker exec
The next command is a cool command: docker exec
. Docker exec is sort of like ‘ssh-ing’ into the container. Let’s “jump” into the dettached container running in the background and explore again.
$ docker exec -ti mystifying_yonath bash
bash-4.2# httpd -v
Server version: Apache/2.4.6 (Amazon Linux 2)
Server built: Dec 12 2017 18:43:44
bash-4.2# cat /etc/os-release
NAME="Amazon Linux"
VERSION="2 (2017.12)"
ID="amzn"
ID_LIKE="centos rhel fedora"
VERSION_ID="2"
PRETTY_NAME="Amazon Linux 2 (2017.12) LTS Release Candidate"
ANSI_COLOR="0;33"
CPE_NAME="cpe:2.3:o:amazon:amazon_linux:2"
HOME_URL="https://amazonlinux.com/"
bash-4.2# exit # leave container and go back to your machine
$
You can leave the container with exit or ctrl-D
.
docker stop
For background containers, you usually must stop them before you can remove them. Here’s how you stop them.
$ docker rm mystifying_yonath
Error response from daemon: You cannot remove a running container a2b93575b0215405dce221d6004ed34d46530fa6d956600241de0fe38f5f7a55. Stop the container before attempting removal or force remove
$ docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
a2b93575b021 boltops/docker-tutorial "/usr/sbin/httpd -DF…" 8 minutes ago Up 8 minutes 0.0.0.0:32768->80/tcp mystifying_yonath
$ docker stop mystifying_yonath
mystifying_yonath
$ docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
$
docker rm
Thus far, we started our containers with the --rm
option. This was nice because then we do not have to manually clean up stopped containers. If you do not use the --rm
option, we would have to clean up the stopped or Exited
containers.
Here’s an example of us having to remove the container when we do not provide the --rm
option. From the top:
$ docker run -P -d boltops/docker-tutorial
58697156b762001ea6a6a8565223054a5df2535b8abe335346ec2822afdae335
$ docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
58697156b762 boltops/docker-tutorial "/usr/sbin/httpd -DF…" 5 seconds ago Up 5 seconds 0.0.0.0:32769->80/tcp mystifying_curie
$ docker stop mystifying_curie
mystifying_curie
$ docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
$ docker ps -a
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
58697156b762 boltops/docker-tutorial "/usr/sbin/httpd -DF…" 2 minutes ago Exited (0) 4 seconds ago mystifying_curie
$ docker rm mystifying_curie
mystifying_curie
$ docker ps -a
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
$ docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
$
Here’s a summary of the commands above:
- We started a new container in detached mode without the
--rm
option this time. - We then listed the background running container out with
docker ps
command. - We then stopped it and then tried to list it out with the
docker ps
command but did not see it. This is because stopped containers only show up with thedocker ps -a
command. - We listed it again with the
docker ps -a
command. We see it this time. - We then removed the “Exited” container for good with
docker rm
- We use
docker ps -a
to confirm that it is gone.
docker start
You can start back up stopped containers with docker start
. You likely won’t be using this as much as docker run
:
docker start mystifying_yonath
That covers the basics of the docker lifecycle commands. A really cool thing once you know these basics is now you can pretty much use any docker container on DockerHub or other registries and play with them. This is useful if you want to quickly test different Linux distros like ubuntu, centos, or amazonlinux:2 and throw them away. Welcome to the power of Docker 😁 Remember the words of wisdom from Spiderman: “With Great Power Comes Great Responsibility.”
Registry Commands: Docker Build and Push
We started off with the docker lifecycle commands to frontload the learning material and help you jump right into using Docker. If you want to build and push your own docker image it requires 3 more commands:
docker build
docker images
docker push
To build a docker image, we’ll grab the same example we’ve been using. Here are the summarized commands:
git clone https://github.com/boltops-learn/docker-tutorial
cd dockerfiles/apache
docker build -t boltops/docker-tutorial .
docker images
docker push boltops/docker-tutorial
Note, I’m pushing to the boltops
DockerHub account. You will have to create a DockerHub user account and push to your own username.
Cleanup Commands: Docker Rm
After playing around with Docker you might end up with some container or image clutter. Here are a few commands that will help clean up the clutter.
Remove all containers:
docker rm `docker ps -a -q`
Remove all “Exited” containers:
docker rm $(docker ps -a | grep 'Exited' | awk '{print $1}')
Remove all images matching a name:
docker rmi $(docker images | grep MYNAME_ | awk '{print $3}')
Wrap Up
Okay, I hope that this basic introduction to Docker has helped ease the learning curve. Like git
it takes only knowing a few of common commands to add another powerful tool to your toolbelt.
Tip: One of the things I’ve noticed is that with git
commands there I do not use options often. With Docker, I use options much more often. The options like -ti
and -P
are very important. So that pay attention to docker options.
Lastly, to get more help to you can appending the --help
flag to the commands:
docker --help
docker run --help
Thanks for reading this far. If you found this article useful, I'd really appreciate it if you share this article so others can find it too! Thanks 😁 Also follow me on Twitter.
Got questions? Check out BoltOps.
You might also like
More tools:
-
Kubes
Kubes: Kubernetes Deployment Tool
Kubes is a Kubernetes Deployment Tool. It builds the docker image, creates the Kubernetes YAML, and runs kubectl apply. It automates the deployment process and saves you precious finger-typing energy.
-
Jets
Jets: The Ruby Serverless Framework
Ruby on Jets allows you to create and deploy serverless services with ease, and to seamlessly glue AWS services together with the most beautiful dynamic language: Ruby. It includes everything you need to build an API and deploy it to AWS Lambda. Jets leverages the power of Ruby to make serverless joyful for everyone.
-
Lono
Lono: The CloudFormation Framework
Building infrastructure-as-code is challenging. Lono makes it much easier and fun. It includes everything you need to manage and deploy infrastructure-as-code.