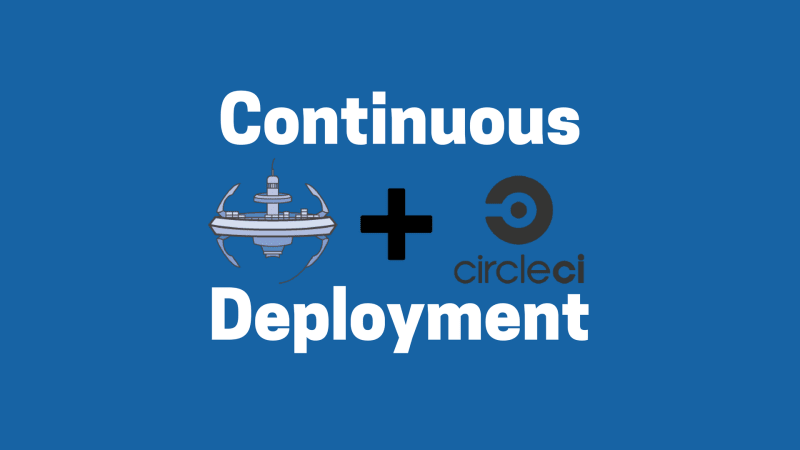
Setting Up Continuous Deployment to ECS on CircleCI
CircleCI is one of my goto hosted Continuous Integration, CI, providers. They make it very easy to quickly connect your GitHub account and set up CI. This story covers how to set up Continuous Deployment to ECS on CircleCI.
Summary of Steps
The example project with complete source code is available at tongueroo/hi in the circle-ecs branch. A few things to note:
-
I’m running tests in Docker containers.
-
When the tests pass then I will use the same exact Docker image used for tests for deployment.
-
I’m using a tool called ufo to ship to ECS.
Test Deployment Locally First
Let’s clone the project and check out the branch to see if you can ship to ECS properly. A thorough guide of how to use ufo is provided here: Easily Build Docker Images and Ship Containers to AWS ECS. Here is a summary of the commands so we can focus on getting the deployment working on CircleCI quickly.
$ git clone [https://github.com/tongueroo/hi.git](https://github.com/tongueroo/hi.git)
$ cd hi
$ git checkout circle-ecs
$ gem install ufo
$ ufo ship hi-web --cluster stag
This deploys the hi-web service to the stag ECS cluster.
Test Deployment on CircleCI
In order to allow CircleCI to deploy to ECS, you need to configure the AWS credentials. Click on the gear icon next to the project name in your project listings:
Save your AWS Key that has ability to deploy to ECS under AWS Permissions:
Add a circle.yml to your project with the following.
machine:
ruby:
version: 2.3.3
services:
- docker
dependencies:
pre:
- gem install --no-ri --no-rdoc ufo
override:
- cp .env.example .env
database:
override:
- cat /dev/null
test:
override:
- ufo docker build
- docker tag $(ufo docker image_name) hi_web
- docker-compose run web bundle exec rake db:create db:migrate
- docker-compose run web bundle exec rake
deployment:
staging:
branch: circle-ecs
commands:
- ufo ship hi-web --cluster stag --no-docker
The dependencies/pre, test/override and the deployment/staging sections are the important ones to cover.
In the dependencies/pre section, the ufo tool is installed.
In the test/override section, the ufo tool is used to to build the docker container because it will generate a Docker image with a nice timestamped tag so we know exactly what image was deployed. Here’s an example of the ufo docker build command in action:
$ ufo docker build
Building docker image with:
docker build -t tongueroo/hi:ufo-2016-12-04T17-18-15-1e9ff5a -f Dockerfile .
Successfully built 820b7b3d5e2a
Docker image tongueroo/hi:ufo-2016-12-04T17-18-15-1e9ff5a built. Took 3s.
$
Right after the docker image is built, I use docker tag $(ufo docker image_name) hi_web to create another tag of hi_web that points to the same timestamped tag name created by ufo docker build. I do this because the docker-compose command will build an image named hi_web when it’s running the tests. This is just to trick the docker-compose command to not build another docker image but reuse the docker image that was built by ufo.
In the deployment/staging section, the branch option is set to circle-ecs because that is the branch which I have the test code in. You should change it for your purposes. You can see that the deployment command is simply: ufo ship hi-web –cluster stag –no-docker. The –no-docker option is provided to ufo so that ufo ship will use the last Docker image that was built by ufo instead of generating a new one. This ensures that we deploy the exact same image that was tested successfully to the ECS service. Now onto verification. For all the options for ufo ship use ufo help ship.
Verify Deployment of CircleCI to ECS
Verify by pushing the project with the circle.yml file to GitHub.
$ git add .
$ git commit -m 'whatever'
$ git push
You should see the build run and at the end of the build the ECS deployment go through. If you have trouble getting CircleCI to deploy, try to ssh into the machine to debug it. Using ssh on CircleCI to debug covered in detailed here: Continuous Integration Provider Killer Debugging Feature: ssh login.
Let’s verify that the Docker image tag that was deployed on ECS matches the one that was generated in the CircleCI build.
You can verify from the ECS Console and check the Task Definition that was deployed to the ECS hi-web service.
You can see that the task definition that was deployed to ECS matches the one that was generated in the CircleCI build, both are tongueroo/hi:ufo-2016–12–05T02–11–43–833c670.
That is all you have to do in order to get deployments to ECS via CircleCI. CircleCI also provides their own documentation on how to deploy to ECS. Hope this helps!
Thanks for reading this far. If you found this article useful, I'd really appreciate it if you share this article so others can find it too! Thanks 😁 Also follow me on Twitter.
Got questions? Check out BoltOps.
You might also like
More tools:
-
Kubes
Kubes: Kubernetes Deployment Tool
Kubes is a Kubernetes Deployment Tool. It builds the docker image, creates the Kubernetes YAML, and runs kubectl apply. It automates the deployment process and saves you precious finger-typing energy.
-
Jets
Jets: The Ruby Serverless Framework
Ruby on Jets allows you to create and deploy serverless services with ease, and to seamlessly glue AWS services together with the most beautiful dynamic language: Ruby. It includes everything you need to build an API and deploy it to AWS Lambda. Jets leverages the power of Ruby to make serverless joyful for everyone.
-
Lono
Lono: The CloudFormation Framework
Building infrastructure-as-code is challenging. Lono makes it much easier and fun. It includes everything you need to manage and deploy infrastructure-as-code.